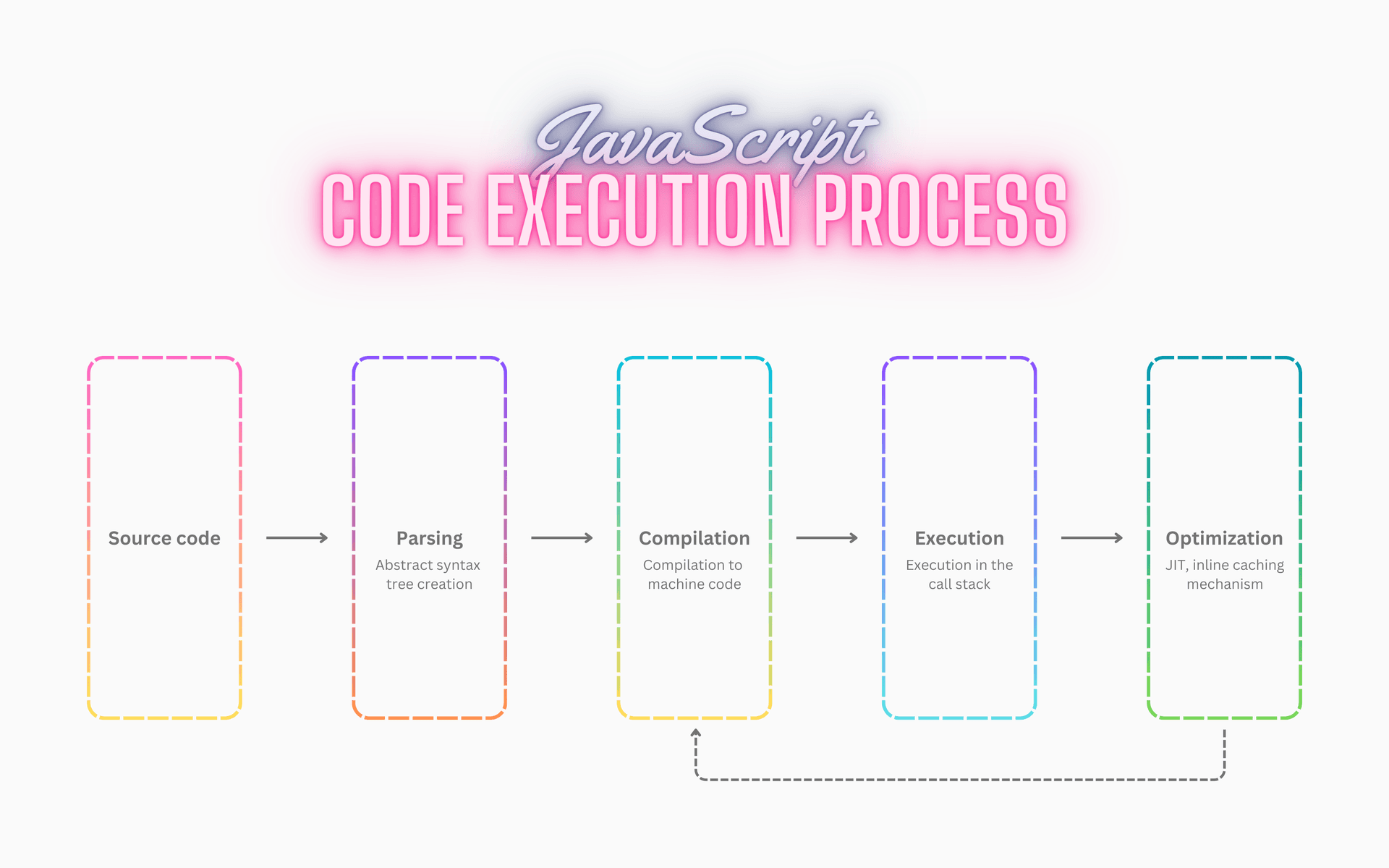
In this article, we will take a close look at how JavaScript code is executed within a JavaScript engine. There are four major steps in the JavaScript code execution process: parsing, compilation, execution, and optimization. Let's take a look at each of these phases in detail.
Parsing
When you run a script, your source code gets parsed by a parser. During parsing, the code is transformed into a data structure called the abstract syntax tree (AST), which represents the structure and semantics of the code. During the creation of the AST, the engine checks for any syntax error in the code.
Compilation
Once the AST is created, a compiler compiles the AST to byte code. Some compilers compile the code directly into machine code. Once the machine code is generated, the engine creates execution contexts to execute the code in the call stack.
A global execution context (GEC) is created for each script, and function execution contexts (FECs) are created for each function in a script.
Before we proceed to the next stage of code execution, let's learn about a few things that happen during the creation of an execution context.
Creation of variable environment and hoisting
The creation of an execution context starts by creating a variable environment. In this environment, properties are created and initialized with different values (based on how variables, functions, and classes are created in the code) for all variables and functions in a script.
These properties are used to achieve the hoisting behavior in JavaScript. In this phase, the arguments
keyword is also generated for each function, containing all the arguments provided to that function.
If you are interested, I have an article on Understanding the variable environment and hoisting in JavaScript that provides further insights.
Creation of scope and scope chain
The next step in execution context creation is the creation of scope and scope chain. Scope and scope chain are necessary to determine which variable is available where in the code.
Scope refers to the environment where variables and functions are created, and scope chain refers to the sequence of connections that is created when a child scope accesses variables from its parent scopes.
I recommend checking out the article on Exploring the scope and scope chain in JavaScript if you are interested in learning more about this topic.
Assignment of the value of the ‘this’ keyword
The final stage in execution context creation is the assignment of the value of the this
keyword. A specific value is assigned to the this
keyword depending on how and where it's used in an execution context.
Read this article on Understanding the dynamic nature of the ‘this’ keyword in JavaScript to deepen your knowledge of this advanced JavaScript topic further.
Execution
Once the execution contexts are created, the JavaScript engine starts the execution within the call stack. The engine places the FEC that is created for the first function call in a script onto the call stack. The call stack then executes and removes the FEC from the stack.
After that, the next FEC is placed onto the call stack and undergoes the same execution process. This pattern of pushing FECs onto the call stack and popping them off continues until all the FECs in a script are executed.
Check out this article on Exploring the inner workings of a JavaScript runtime to learn more about how synchronous, asynchronous, and callback functions are executed in a JavaScript runtime.
Optimization
After several initial compilation and execution cycles, the JavaScript engine starts optimizing the code. It checks for the functions that are running frequently with the same data types.
The engine optimizes those functions and recompiles the code. Optimization techniques like just-in-time compilation (JIT) and inline caching are used to optimize and run the code faster.
There you have it! I hope you now have a better understanding of the JavaScript code execution process and how it works behind the scenes.